if(Chunk)
{
world_entity_block *FirstBlock = &Chunk->FirstBlock;
for(world_entity_block *Block = FirstBlock;
Block;
Block = Block->Next)
{
for(uint32 Index = 0;
Index < Block->EntityCount;
++Index)
{
if(Block->LowEntityIndex[Index] == LowEntityIndex)
{
Assert(FirstBlock->EntityCount > 0);
Block->LowEntityIndex[Index] =
FirstBlock->LowEntityIndex[--FirstBlock->EntityCount];
// At this point the first block is empty and needs to be reclaimed
if(FirstBlock->EntityCount == 0)
{
// If the firstblock has a next block because otherwise you don't need to reclaim it
if(FirstBlock->Next)
{
// make a fresh pointer to the next block of first
world_entity_block *NextBlock = FirstBlock->Next;
// copy the contents of the next block into the first block
*FirstBlock = *NextBlock;
// Set the next block into the list of free blocks
NextBlock->Next = World->FirstFree;
// Set the head of freeblocks to be this block
World->FirstFree = NextBlock;
}
}
Block = 0;
break;
}
}
}
}
Explained with images it goes like this. So from right to left imagine that the green line is your memory:
- make a pointer N.B. (in pink) to the next of the first block F.B.
- Copy all contents of N.B. to the memory of F.B. This will also copy the next pointer of N.B. to F.B. (sorry for bad drawing), and now both N.B. and F.B. have next pointers pointed to the unnamed next block of the next block as pictured.
- Move the next pointer of the N.B. to the firstfreeblock which is basically a collection of memory laying around to be used later
- Update the first free pointer (F.F.) to be this N.B.
So you end up with the next block's contents in the first block and the nextblock itself becomes discarded.
So clever! I might be wrong though as I'm new to this stuff as well.
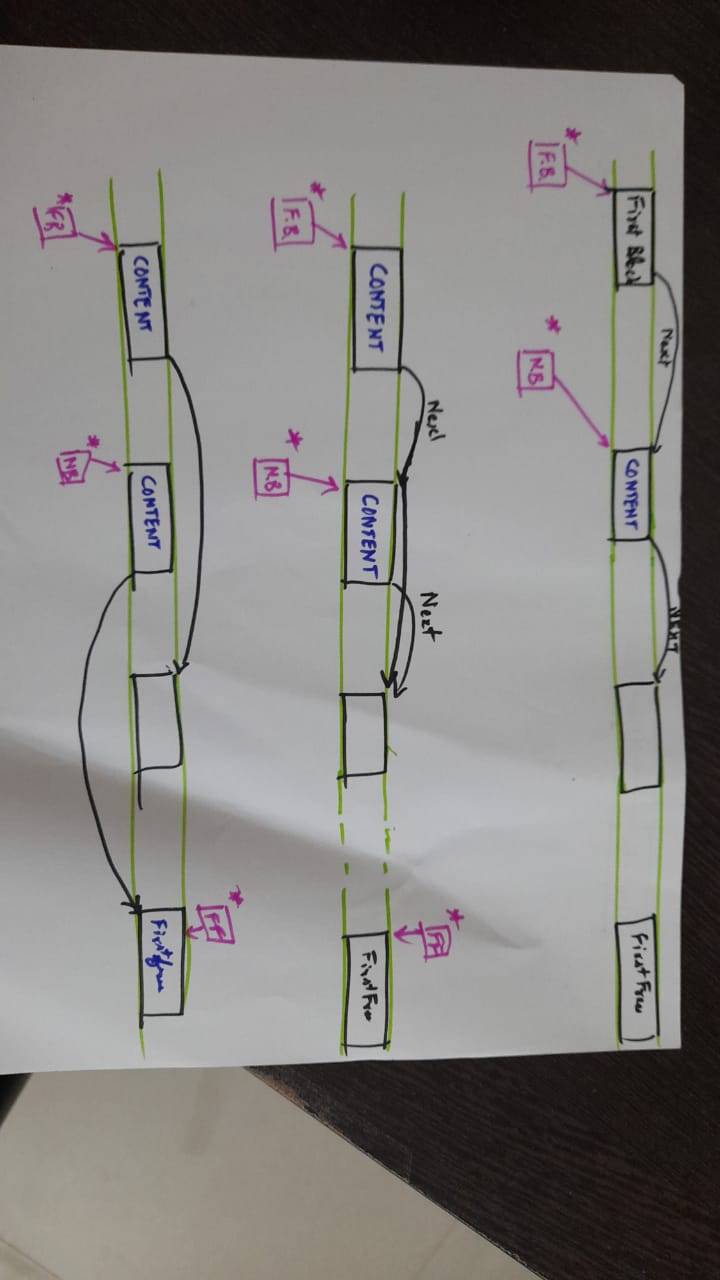