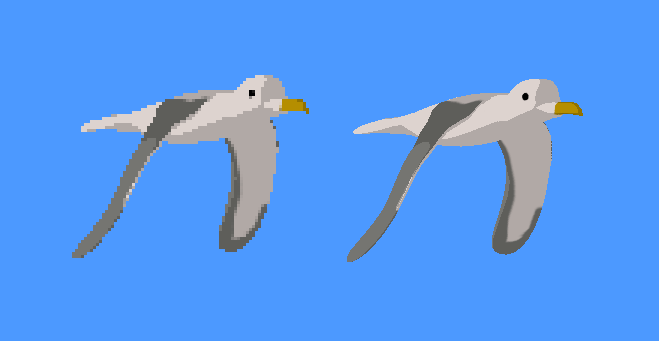

(It is easier to see the difference if you open the image in a new window to avoid the page from scaling the image.)
On the left is an image of a bird flying in the distance, rendered without the program being High DPI aware. This is running on Windows 10 with a 300% desktop scale on a 3840x2160 (4K) resolution laptop monitor. On the right is the exact same rendering, except rendered with High DPI awareness. There is a noticeable increase in resolution due to the application being able to utilize all of the pixels that the model is occupying on the display for graphics fragment processing.
Even worse than losing potential pixels is the fact that the output image of a non-aware application is scaled by Windows when the display scale is set to any value above 100%. This scaling might not seem like a problem, but due to the user being able to set the desktop scale in 25% increments, potentially unappealing bilinear scaling will result. This is especially problematic for font rendering, as it is critical for the image clarity of the font that it is not scaled either larger or smaller after initial rasterization before being written to the screen.
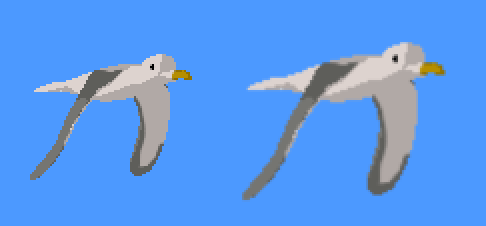

On the left is a non-aware rendering done with a desktop scale of 100%. On the right is also a non-aware rendering but with a desktop scale of 125%. It might be difficult to see depending on your monitor, but the image on the right contains an outline of blurred pixels all around the edges of the model.
I noticed this when I ran Seabird on a different computer than the one I had previously used for development. The rendered image quality on the new computer appeared to be significantly lower than it should have been given the resolution of screen I was using. I became especially suspicious discovering that other desktop application such as my browser and office suite did not suffer from this quality problem. This prompted further investigation.
On High DPI monitors the number of pixels per inch can far exceed 140, which is greater than the 96 pixels per inch that Windows assumes by default. The motivation for designing screens with a higher pixel density instead of larger physical dimensions I believe was the smartphones and tablet devices. It is not uncommon to see someone hold a smartphone or tablet 12 inches from their eyes. This necessitated screens which could convey the same visual information as a desktop monitor or TV screen, while being constrained to a screen with a surface area measured in tens of inches instead of hundreds of inches.
One thing to be aware of when writing code a High DPI aware application is that you might need to read the desktop scale from the operating system in order to correctly handle scaling in your application. High DPI aware applications are also supposed to be able to dynamically respond to desktop scale change events.
Here is a short example for the minimum code needed to enable High DPI awareness when using the SDL library on Windows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | #pragma comment(lib, "Shcore.lib"); #include <windows.h> #include <ShellScalingAPI.h> #include <comdef.h> #include "SDL.h" int main(int argc, char *argv[]) { SetProcessDpiAwareness(PROCESS_PER_MONITOR_DPI_AWARE); SDL_Window *window = SDL_CreateWindow( "High DPI aware SDL window", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, 1000, 1000, SDL_WINDOW_ALLOW_HIGHDPI /* | in other flags here */); return 0; } |
https://nlguillemot.wordpress.com/2016/12/11/high-dpi-rendering/
https://msdn.microsoft.com/en-us/...ary/windows/desktop/hh447398.aspx